In this section, we are going to learn the use html view file so that we can generate PDF. Suppose we are working on a big ERP level laravel project. In this case, we need to use a database table to generate a PDF file for the required data. We will provide a very simple step-by-step process to generate pdf file and download it.
Also Read: How To Fix the Elementor Loading Error in WordPress
Now we will create a pdf file by using the laravel-dompdf package. Using this package, we can also download the function. In the below example, we will create a table named “items”. We will use the data of item table to download pdf file of it in tabular format. If we want, we can generate a better pdf file by writing our CSS on the view file. Lastly, we will generate a pdf and download it by using some steps, which is as follows. For this, we should have a fresh and working project of Laravel 5 or Laravel 5.3, etc.
Step 1:
In this step, we will do Installation. We will firstly open our terminal or command prompt and run the following command:
composer require barryvdh/laravel-dompdf
Now we will open our file named config/app.php. Then we will add aliased and service provider.
'providers' => [
....
Barryvdh\DomPDF\ServiceProvider::class,
],
'aliases' => [
....
'PDF' => Barryvdh\DomPDF\Facade::class,
],
Step 2:
In this step, we will Add Route. We will create route so that we can generate a view. So we will open our file named “app/Http/routers.php”. After that, we will add the following route:
Route::get('pdfview',array('as'=>'pdfview','uses'=>'ItemController@pdfview'));
Step 3:
In this step, we will Create Controller. For this, we are going to use the app/Http/Controllers/ItemController.php path to add a new controller as ItemController. If we want to perform this, we require an item table that will contain some data. When we want to generate a pdf file and manage table data, this controller will be useful. So we will use the controller file and put the following code into it:
app/Http/Controllers/ItemController.php
namespace App\Http\Controllers;
use App\Http\Requests;
use Illuminate\Http\Request;
use DB;
use PDF;
class ItemController extends Controller
{
/**
* It will display application dashboard.
*
* It will return \Illuminate\Http\Response
*/
public function pdfview(Request $request)
{
$items = DB::table("items")->get();
view()->share('items',$items);
if($request->has('download')){
$pdf = PDF::loadView('pdfview');
return $pdf->download('pdfview.pdf');
}
return view('pdfview');
}
}
Step 4:
In this step, we will Create View file. We will create view and pdf file by using the view file named as “pdfview.blade.php”. So we will add the following code in created pdfview file.
resources/view/pdfview.blade.php
<style type="text/css">
table td, table th{
border:1px solid black;
}
</style>
<div class="container">
<br/>
<a href="{{ route('pdfview',['download'=>'pdf']) }}">Download PDF</a>
<table>
<tr>
<th>No</th>
<th>Name</th>
<th>Email</th>
</tr>
@foreach ($items as $key => $item)
<tr>
<td>{{ ++$key }}</td>
<td>{{ $item->name }}</td>
<td>{{ $item->email }}</td>
</tr>
@endforeach
</table>
</div>
Using the above code we are able to convert item file into pdf file and also able to download that file. In order to run the above code, we need to start the server of Laravel 5 or Laravel 5.3. For this, we will open our terminal or command prompt and run the following command:
php artisan serve
Using the above command, we are able to start the Laravel server. It will provide us base url of the Laravel application. We will open our browser and write the following url to test the above code.
http://127.0.0.1:8000/pdfview
After entering the above URL, we will see the following output:
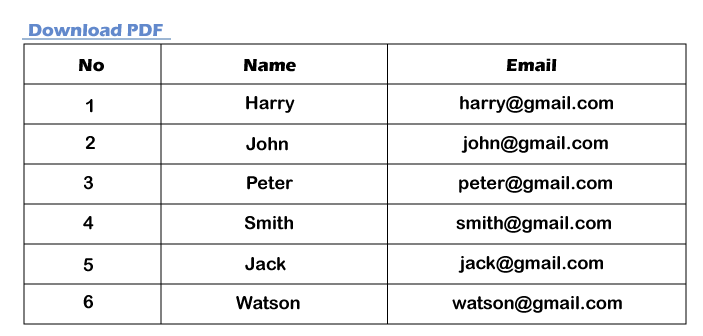